Exploring React Hook Form for Efficient Form Handling in React Applications
by Jessy E Jose (Junior Software Developer) | Updated on August 16, 2024
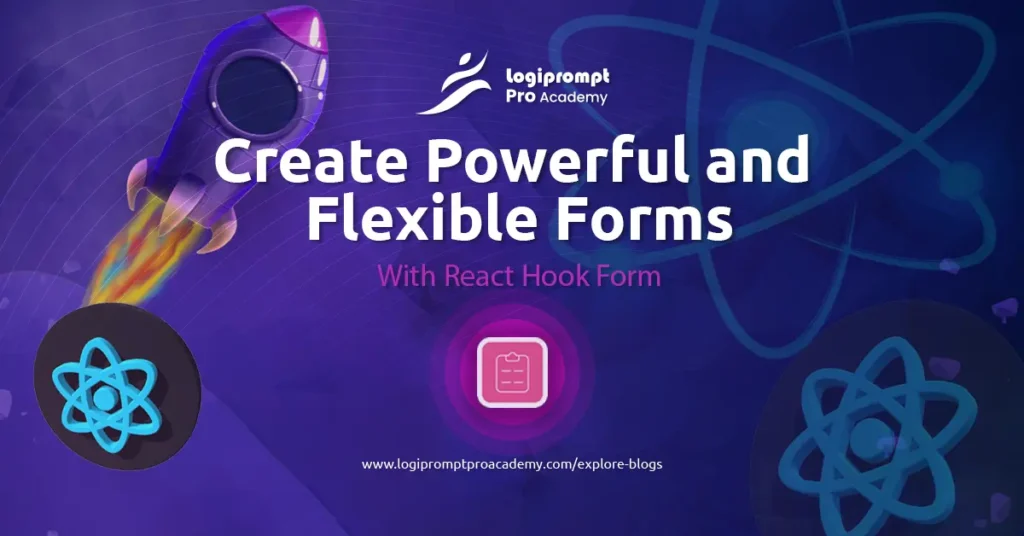
As a passionate developer, I’ve always sought to create efficient and user-friendly applications. In modern web development, creating forms that are efficient, maintainable, and user-friendly is crucial. React, with its robust ecosystem and libraries, provides several tools to achieve this. One such powerful tool is react-hook-form, which simplifies form handling in React applications by leveraging React hooks.
What is react-hook-form?
React-hook-form is a library that optimizes form management in React by using hooks such as useForm, useController, and more. It promotes a performance-oriented approach by minimizing re-renders and providing efficient form validation and submission handling.
Key Features of React-hook-form
Performance Optimization Unlike traditional React form libraries that may cause unnecessary re-renders, react-hook-form optimizes performance by minimizing rerenders and improving the overall efficiency of form handling.
Hook-based Approach Built entirely on React hooks, react-hook-form simplifies form management without the need for complex class components or higher-ordercomponents (HOCs). Hooks such as useForm, useController, useFieldArray, etc., provide a concise and intuitive API for managing form state and validation.
Minimal Footprint With a small footprint, react-hook-form reduces bundle size and improves load times, making it suitable for projects where performance and speed are critical considerations.
Integration with UI Libraries react-hook-form seamlessly integrates with popular UI libraries and components like Material-UI, Ant Design, React-Select, etc., making it versatile and adaptable to various design systems.
Efficient Validation It supports both built-in and custom validation rules, allowing developers to validate form inputs easily. Validation errors are handled efficiently and can be displayed to users with minimal effort.
Setting Up the Project
Here’s a brief overview of my setup process:
- Create a React Application: I started by setting up a new React project using createreact-app
- Install react-hook-form: I added react-hook-form to my project using npm.
npm install react-hook-form - Additional Dependencies: Depending on my project requirements, I need other
libraries like axios for making API requests, react-router-dom for routing, and so on
Implementing the Registration Form
Let’s walk through the implementation of a student registration form using react-hook-form. This example assumes familiarity with basic React concepts like state management, event handling, and JSX syntax.
Setting Up the Form
import React from 'react';
import { useForm } from 'react-hook-form';
const Register = () => {
const {register,handleSubmit,formState: { errors }} =
useForm();
const onSubmit = (data) => {
// Handle form submission
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
{/* Form fields */}
<input
type="text"
placeholder="Enter your name"
{...register('name', { required: 'Name is required' })}
/>
{errors.name && (
<span role="alert" className="error">
{errors.name.message}
</span>
)}
<input type="submit" />
</form>
);
};
export default Register;
Implementing Form Fields
Using react-hook-form,we can simplifies the integration of form fields with minimal code:
<input
type="text"
placeholder="Enter your name"
{...register('name', {
required: 'Name is required',
minLength: {
value: 2,
message: 'Name should have at least 2 characters',
},
})}
/>
{errors.name && (
<span role="alert" className="error">
{errors.name.message}
</span>
)}
Handling Form Submission
We have handle form submission with handleSubmit:
const onSubmit = (data) => {
// Handle form submission, e.g., send data to backend
console.log(data);
};
Integrating Select Components
Integrate dropdowns using react-select and Controller:
import Select from 'react-select';
import { Controller, useForm } from 'react-hook-form';
const Register = () => {
const { control } = useForm();
return (
<Controller
name="country"
control={control}
render={({ field }) => (
<Select
{...field}
options={[
{ value: 'usa', label: 'United States' },
{ value: 'canada', label: 'Canada' },
]}
/>
)}
/>
);
};
export default Register;
Custom Validation with Patterns and Error Handling
React-hook-form makes it easy to handle custom validation using patterns (regex) and display
error messages accordingly. Here’s how I implemented custom validation for an email field:
<div className="col-6">
<label className="col-form-label pt-0">Email Address</label>
<input
type="email"
className={`form-control ${errors.email ? 'is-invalid' :
''}`}
id="email"
placeholder="name@trail.com"
{...register('email', {
required: 'Email is required',
pattern: {
value:/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zAZ]{2,}$/,
message: 'Please enter a valid email address',
},
})}
/>
{errors.email && (
<div className="invalid-feedback">
{errors.email.message}
</div>
)}
</div>
Conclusion
In this tutorial, I explored react-hook-form, a powerful library for managing forms in React applications efficiently. I covered its key features, setup process, and implementation steps for building a registration form. Additionally, I demonstrated how to implement custom validation using patterns (regex) and handle error messages effectively. By leveraging react-hook-form, developers can streamline form development, improve performance, and enhance user experience in their React projects.
Explore & Learn
Embark on a journey of knowledge and discovery with our curated collection of articles, insights, and updates to foster continuous learning and exploration.